- Creating and accessing list
- List operation
- Working with list
- List Function and Method
What is Python List ?
- Python offers a range of compound data types often referred to as sequences.
- List is one of the most frequently used and very versatile data types used in Python
How to create a list?
- In Python programming, a list is created by placing all the items (elements) inside square brackets
[]
, separated by commas. - It can have any number of items and they may be of different types (integer, float, string etc.)
# empty list
list = []
# list of integers
list = [1, 2, 3]
# list with mixed data types
list = [1, "Hello", 3.4]
What is nested List ?
- A list can also have another list as an item. This is called a nested list
# nested list
list = ["mouse", [8, 4, 6], ['a']]
How to access elements from a list?
There are various ways in which we can access the elements of a list.
1.List Index
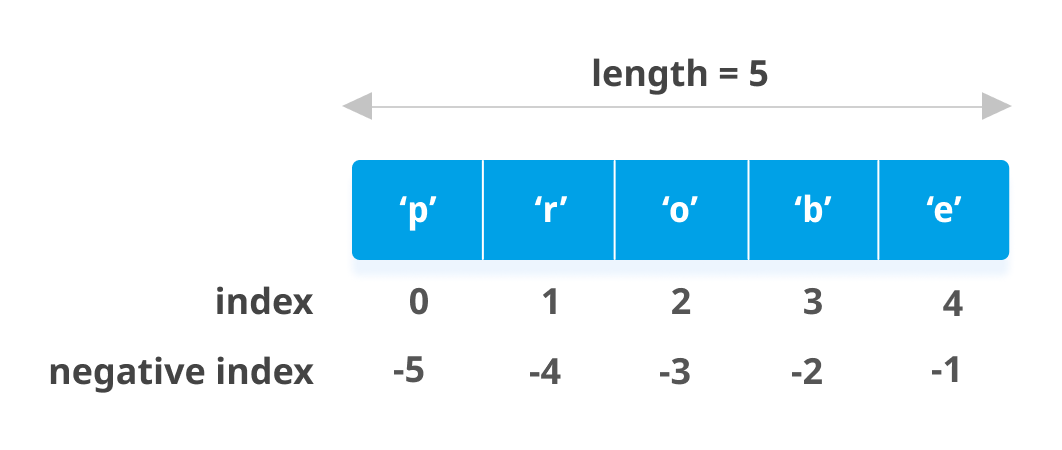
- We can use the index operator
[]
to access an item in a list. In Python, indices start at 0. So, a list having 5 elements will have an index from 0 to 4. - Trying to access indexes other than these will raise an
IndexError
. The index must be an integer. We can't use float or other types, this will result inTypeError
. - Nested lists are accessed using nested indexing.
# List indexing
list = ['p', 'r', 'o', 'b', 'e']
# Output: p
print(list[0])
# Output: o
print(list[2])
# Output: e
print(list[4])
# Nested List
n_list = ["Happy", [2, 0, 1, 5]]
# Nested indexing
print(n_list[0][1])
print(n_list[1][3])
# Error! Only integer can be used for indexing
print(list[4.0])
Output
p
o e a 5 Traceback (most recent call last): File "<string>", line 21, in <module> TypeError: list indices must be integers or slices, not float
2.Negative indexing
- Python allows negative indexing for its sequences. The index of -1 refers to the last item, -2 to the second last item and so on.
# Negative indexing in lists
list = ['p','r','o','b','e']
print(list[-1])
print(list[-5])
When we run the above program, we will get the following output:
e p
How to slice lists in Python?
We can access a range of items in a list by using the slicing operator:
(colon).
# List slicing in Python
list = ['p','r','o','g','r','a','m','i','z']
# elements 3rd to 5th
print(list[2:5])
# elements beginning to 4th
print(list[:-5])
# elements 6th to end
print(list[5:])
# elements beginning to end
print(list[:])
Output
['o', 'g', 'r'] ['p', 'r', 'o', 'g'] ['a', 'm', 'i', 'z'] ['p', 'r', 'o', 'g', 'r', 'a', 'm', 'i', 'z']
How to change or add elements to a list?
We can use the assignment operator (
=
) to change an item or a range of items.
# Correcting mistake values in a list
odd = [2, 4, 6, 8]
# change the 1st item
odd[0] = 1
print(odd)
# change 2nd to 4th items
odd[1:4] = [3, 5, 7]
print(odd)
Output
[1, 4, 6, 8] [1, 3, 5, 7]
List Comprehension: Elegant way to create new List
- List comprehension is an elegant and concise way to create a new list from an existing list in Python.
- A list comprehension consists of an expression followed by for statement inside square brackets.
- A list comprehension can optionally contain more
for
or if statements. An optionalif
statement can filter out items for the new list. - Here is an example to make a list with each item being increasing power of 2.
pow2 = [2 ** x for x in range(10)]
print(pow2)
Output
[1, 2, 4, 8, 16, 32, 64, 128, 256, 512]
>>> pow2 = [2 ** x for x in range(10) if x > 5]
>>> pow2
[64, 128, 256, 512]
>>> odd = [x for x in range(20) if x % 2 == 1]
>>> odd
[1, 3, 5, 7, 9, 11, 13, 15, 17, 19]
>>> [x+y for x in ['Python ','C '] for y in ['Language','Programming']]
['Python Language', 'Python Programming', 'C Language', 'C Programming']
Operation on list
- We can also use
+
operator to combine two lists. This is also called concatenation. - The
*
operator repeats a list for the given number of times.
# Concatenating and repeating lists
odd = [1, 3, 5]
print(odd + [9, 7, 5])
print(["pre"] * 3)
Output
[1, 3, 5, 9, 7, 5] ['pre', 'pre', 'pre']
- List Membership Operator
We can test if an item exists in a list or not, using the keyword in
.
my_list = ['p', 'r', 'o', 'b', 'l', 'e', 'm']
# Output: True
print('p' in my_list)
# Output: False
print('a' in my_list)
# Output: True
print('c' not in my_list)
Output
True False True
Iterating Through a List
Using a for
loop we can iterate through each item in a list.
for fruit in ['apple','banana','mango']:
print("I like",fruit)
Output
I like apple I like banana I like mango
Python List Methods
- Methods that are available with list objects in Python programming are tabulated below.
- They are accessed as
list.method()
0 comments:
Post a Comment