What is String in Python?
A string is a sequence of characters.A character is simply a symbol. For example, the English language has 26 characters
In Python, a string is a sequence of Unicode characters. Unicode was introduced to include every character in all languages and bring uniformity in encoding. You can learn about Unicode from Python Unicode.
Strings are immutable. This means that elements of a string cannot be changed once they have been assigned.
How to create a string in Python?
# defining strings in Python
mystring = 'Hello'
print(mystring)
mystring = "Hello"
print(mystring)
mystring = '''Hello'''
print(mystring)
# triple quotes string can extend multiple lines
mystring = """Hello, welcome to
the world of Python"""
print(mystring)
Hello Hello Hello, welcome to the world of Python
How to access characters in a string?
String Indexing |
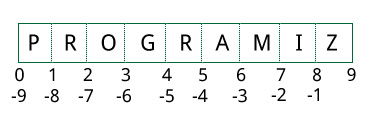
IndexError
. The index must be an integer. We can't use floats or other types, this will result into TypeError
.-1
refers to the last item, -2
to the second last item and so on. We can access a range of items in a string by using the slicing operator :
(colon).#Accessing string characters in Python
str = 'programiz'
print('str = ', str)
#first character
print('str[0] = ', str[0])
#last character
print('str[-1] = ', str[-1])
#slicing 2nd to 5th character
print('str[1:5] = ', str[1:5])
#slicing 6th to 2nd last character
print('str[5:-2] = ', str[5:-2])
str = programiz str[0] = p str[-1] = z str[1:5] = rogr str[5:-2] = am
Python String Operations
There are many operations that can be performed with string which makes it one of the most used data types in Python.
Concatenation of Two or More Strings
Joining of two or more strings into a single one is called concatenation.
The + operator does this in Python. Simply writing two string literals together also concatenates them.
The * operator can be used to repeat the string for a given number of times.
# Python String Operations
str1 = 'Hello'
str2 ='World!'
# using +
print('str1 + str2 = ', str1 + str2)
# using *
print('str1 * 3 =', str1 * 3)
str1 + str2 = HelloWorld! str1 * 3 = HelloHelloHello
>>> # using parentheses
>>> s = ('Hello '
... 'World')
>>> s
'Hello World'
String Membership Test
We can test if a substring exists within a string or not, using the keyword in
.
>>> 'a' in 'program'
True
>>> 'at' not in 'battle'
False
Iterating or Traversing Through a string
We can iterate through a string using a for loop. Here is an example to count the number of 'l's in a string.
# Iterating through a string
count = 0
for letter in 'Hello World':
if(letter == 'l'):
count += 1
print(count,'letters found')
When we run the above program, we get the following output:
3 letters found
Raw String to ignore escape sequence
Sometimes we may wish to ignore the escape sequences inside a string. To do this we can place r
or R
in front of the string. This will imply that it is a raw string and any escape sequence inside it will be ignored.
>>> print("This is \x61 \ngood example")
This is a
good example
>>> print(r"This is \x61 \ngood example")
This is \x61 \ngood example
Common Python String Methods
There are numerous methods available with the string object. The format()
method that we mentioned above is one of them. Some of the commonly used methods are lower()
, upper()
, join()
, split()
, find()
, replace()
etc. Here is a complete list of all the built-in methods to work with strings in Python.
>>> "PrOgRaMiZ".lower()
'programiz'
>>> "PrOgRaMiZ".upper()
'PROGRAMIZ'
0 comments:
Post a Comment